How to fix the Unbound module Graphics in an ocaml project
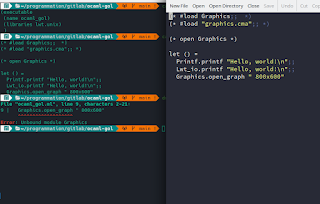
RainbruRPG is an open-source MMORPG project I'm working on since someday in early 2010's. I already posted multiple posts on this project (for example here and here). While its development is actually in pause for a while now, it is a great project to learn using new 3D/UI oriented libraries. CEGUI (short for Crazy Eddie's GUI System) is one of these libraries. It is a free MIT-licensed windowing and widgets library that run on top of Ogre3D but it can also run on other backends.
![]() |
The RainbruRPG's not yet implemented modal dialog |
ModalDialog md; if (md.exec()) { // handle positive event }
In the above example, the code is blocked in the exec()
until the end user click on a button or close the dialog.
Here is the header sources but it is mainly based on another class (called CeguiDialog I will not include here. You can find it online at bitbucket. You may want to refer at least to its header because it contains some members and include needed here (mDialogWindow, mDialog etc...) :
#include <CEGUI/EventArgs.h> // Used as a reference #include "CeguiDialog.hpp" // Forward declarations namespace Ogre { class Root; } class GameEngine; // End of forward declarations using namespace std; class ModalDialog : public CeguiDialog { public: ModalDialog(const string&, const string&, const string&, const string&); ~ModalDialog(); bool exec(GameEngine*); protected: bool onOk(const CEGUI::EventArgs&); bool onCancel(const CEGUI::EventArgs&); bool closeDialog(bool); private: bool waiting; bool returnValue; };
bool exec(GameEngine*) { // This boolean must be set to false by a CEGUI slot, for // example the Ok or Cancel buttons slot waiting = true; // Shows the dialog mDialogWindow->setVisible(true); mDialog->setVisible(true); mDialog->activate(); while (waiting) { // Tested to make the the GeometryBuffer object appear CEGUI::System::getSingleton().getDefaultGUIContext(). clearGeometry(CEGUI::RQ_OVERLAY); // Make Ogre3D render one frame Ogre::WindowEventUtilities::messagePump(); root->renderOneFrame(); } return returnValue; }
void closeDialog(bool value) { waiting = false; hide(); // Set the return value of the dialog returnValue = value; } bool CeguiDialog::onOk(const CEGUI::EventArgs&) { closeDialog(true); // Tells CEGUI we fully handled the event return true; } CEGUI::PushButton* btnOk = (CEGUI::PushButton *)mDialogWindow-> getChild("nyiRoot/winToolbar/btnOk"); btnOk->subscribeEvent(CEGUI::PushButton::EventClicked, CEGUI::Event::Subscriber(&CeguiDialog::onOk, this));
bool CeguiDialog::onCancel(const CEGUI::EventArgs&) { closeDialog(false); // Tells CEGUI we fully handled the event return true; } CEGUI::PushButton* btnCancel = (CEGUI::PushButton *)mDialogWindow-> getChild("nyiRoot/winToolbar/btnCancel"); btnCancel->subscribeEvent(CEGUI::PushButton::EventClicked, CEGUI::Event::Subscriber(&CeguiDialog::onCancel, this));
This modal dialog, in its current state, event if not perfect, works. If I manage to improve it, I'll try to update this article.
Comments
Post a Comment