How to fix the Unbound module Graphics in an ocaml project
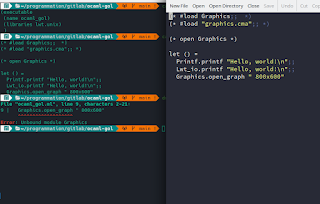
A singleton is a class that can only be instantiated once. The design pattrern restricts the class to one single instance and further access will always return the same, first one.
As explained in the singleton.html page, the boost serialization library has a Singleton class defined in the shipped Singleton.hpp C++ header.
On arch-based distributions, such as manjaro, this header is part of the extra/boost package. On Debian Bullseye, it is part of the libboost1.74-dev one but it's a dependency of the libboost-dev dependency package. So, according to your distribution, use the needed command :
sudo pacman -S boost or sudo apt-get install libboost-dev
As seen in the boost class interface documentation, to use it, you just have to create a class that inherits the singleton one.
Here is an example class that use boost's singleton to ensure one and only one instance will be in use :
#include <boost/serialization/singleton.hpp> using namespace boost::serialization; /** Here is the GameEngine singleton * */ class GameEngine : public singleton<GameEngine> { public: GameEngine(){}; GameEngine(const GameEngine&){}; };
Now you can get the unique instance using either
GameEngine::get_const_instance()to get a constant (i.e. non-modifiable) instance or
GameEngine::get_mutable_instance()to get a mutable one.
As always, cmake can be usefull to search for the presence of the library :
find_package(Boost REQUIRED COMPONENTS serialization)
And even if boost serialization is a header only library, you don't need to add it to linker flags but if you need it for another boost component you should use :
target_link_libraries(your-target-name ${Boost_LIBRARIES})
The boost serialization library obviously implements many more than a simple singleton class. Fore more, you can see the reference, case studies or other classes sections of the official documentation.
You may also be interested by the multi-thread usage of the singleton class. Basically, you have to call these functions :
void boost::serialization::singleton_module::lock(); void boost::serialization::singleton_module::unlock(); bool boost::serialization::singleton_module::is_locked();
More can be read here in the official documentation.
Comments
Post a Comment