How to fix the Unbound module Graphics in an ocaml project
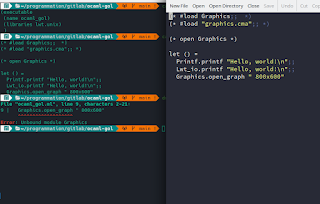
From ~/pr/gitl/ocaml-gol In a constant effort to learn new programming languages, I'm currently trying to use ocaml , a free and open-source general-purpose, multi-paradigm programming language maintained at the Inria . It's basically an extension of Caml with object-oriented features. I'm mostly interested by its functionnal and pattern matching features but the module part of the language can be a bit difficult to understand for someone with little to none ML (Meta Language) background. The error When trying to use the graphics module to create a graphical window and go just a little further than the simplest helloworld program, here is the result : If the project uses dune : (executable (name ocaml_project) (libraries lwt.unix graphics) ) with this code : let () = Printf.printf "Hello, world!\n";; Lwt_io.printf "Hello, world!\n";; Graphics.open_graph " 800x600";; The first times I built this project running the du
Comments
Post a Comment